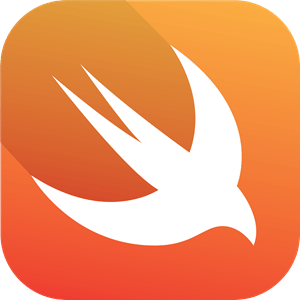
접근제어자란
코드 내의 특정 부분에 대한 접근 권한을 설정하여 코드의 캡슐화를 강화하고 보안을 유지하는데 사용된다.
open
가장 넓은 접근 수준, 모듈 외부에서 해당 요소에 접근하고 하위 클래싱(클래스) 및 재정의(메서드)를 허용한다.
주로 프레임워크나 라이브러리르 설계할 때 외부 모듈에서 상속 및 재정의를 허용하려는 경우 사용된다.
open class OpenClass {
open func openMethod() {}
}
public
모듈 외부에서 접근은 가능하지만, 하위 클래싱 및 재정의는 허용되지 않는다.
주로 외부에서 접근 가능해야 하지만 내부 동작은 수정하지 못하도록 제한하고 싶은 경우 사용된다.
public class PublicClass {
public var publicProperty = "I am public"
public func publicMethod() {
print("This is a public method")
}
}
let instance = PublicClass()
print(instance.publicProperty) // "I am public"
instance.publicMethod() // "This is a public method"
internal(기본 값)
동일 모듈 내에서 접근할 수 있다. 모듈 외부에서는 접근이 불가능하다.
모듈 내부에서만 사용되는 코드에 대한 기본 설정이다.
따로 다른 접근제어자를 설정하지 않고 작성한다면 기본적으로 Internal로 설정된다.
class InternalClass {
var internalProperty = "I am internal"
func internalMethod() {
print("This is an internal method")
}
}
let instance = InternalClass()
print(instance.internalProperty) // "I am internal"
instance.internalMethod() // "This is an internal method"
private
동일한 Scope 및 extension(같은 파일 내) 내에서만 접근할 수 있다.
주로 완전히 외부 노출을 막고, 특정 타입 내부에서만 사용할 수 있도록 제한하려는 경우 사용된다.
class PrivateClass {
private var privateProperty = "I am private"
private func privateMethod() {
print("This is a private method")
}
func accessPrivateProperty() {
print(privateProperty) // "I am private"
privateMethod() // "This is a private method"
}
}
let instance = PrivateClass()
instance.accessPrivateProperty()
// instance.privateProperty // 오류 발생
// instance.privateMethod() // 오류 발생
fileprivate
동일 파일 내에서만 접근할 수 있다.
주로 한 파일 내에서만 사용할 수 있도록 제한하려는 경우 사용된다.
class FileprivateClass {
fileprivate var fileprivateProperty = "I am fileprivate"
fileprivate func fileprivateMethod() {
print("This is a fileprivate method")
}
}
class AnotherClass {
func accessFileprivateProperty() {
let instance = FileprivateClass()
print(instance.fileprivateProperty) // "I am fileprivate"
instance.fileprivateMethod() // "This is a fileprivate method"
}
}
static과 final
static과 final은 접근제한자는 아니다.
특정 동작을 정의하거나 제한하기 위해 사용되는 키워드이다.
접근제어자와는 별개의 개념이지만 함께 사용될 수 있다.
static
static은 타입 자체에 속하는 타입 멤버를 정의할 때 사용된다.
특정 인스턴스에 속하지 않고 클래스, 구조체, 또는 열거형 타입에 직접 속하는 변수, 상수, 또는 메서드를 선언할 때 사용된다.
struct StaticExample {
static var staticProperty = "I am static"
static func staticMethod() {
print("This is a static method")
}
}
print(StaticExample.staticProperty) // "I am static"
StaticExample.staticMethod() // "This is a static method"
struct Math {
static let pi = 3.14159
static func square(_ number: Double) -> Double {
return number * number
}
}
print(Math.pi) // 3.14159
print(Math.square(4)) // 16.0
즉 이런 식으로 인스턴스를 만들지 않아도 바로 사용이 가능하다.
Math.pi
StaticExample.staticProperty
static.staticMethod
final
클래스, 메서드, 프로퍼티 등의 상속 및 재정의를 할 수 없도록 한다.
final은 상속과 관련된 제약을 설정하기 때문에 주로 클래스에서 사용된다.
클래스에 적용시 - 상속을 아예 금지함. 해당 클래스는 서브클래스를 가질 수 없다.
메서드 / 프로퍼티 / 서브스크립트에 적용시 - 하위 클래스에서 해당 멤버를 재정의할 수 없다.
final class FinalClass {
final var finalProperty = "I am final"
final func finalMethod() {
print("This is a final method")
}
}
// FinalClass를 상속받으려 하면 오류 발생
class SubClass: FinalClass {} // 오류 발생
class Vehicle {
final func startEngine() {
print("Engine started")
}
}
// 이 코드는 오류 발생: 'startEngine'은 재정의가 금지됨
class Car: Vehicle {
override func startEngine() { }
}
'Language > Swift' 카테고리의 다른 글
(작성중)Swift 기본 문법 - Enumeration(열거형) (0) | 2024.11.05 |
---|---|
Swift 기본 문법 - 클로저(Closure) 작성중 (0) | 2024.11.01 |
Swift 기본 문법 - Double 타입에서 나머지 구하기(TruncastingRemainder) (0) | 2024.10.29 |
Swift 기본 문법 - Tuple (0) | 2024.10.28 |
Swift 기본 분법 - 반복문 (for문, while문) (0) | 2024.10.20 |
살아남는 iOS 개발자가 되기 위해 끊임없이 노력하고 있습니다.